2D Audio Listener for Music and UI in Unreal Engine and Wwise
Si prefieres leer una versión en Español, da click en este botón =>
Unreal Engine Version: 5.3.2
Wwise Version: 2023.1.0
This blog post shows how to register a 2D (Non-Spatial Audio) listener and a Wwise Object to post Music and UI sounds that don’t require 3D positioning in the Game World. These Wwise objects are registered using an audio Manager and don’t get destroyed on level transitions, which means that audio playback won’t be interrupted. This implementation is not possible using visual scripting, but I show how to expose some of its functionality to Blueprint.
Here is the list of essential tools and keywords used for this project:
I programmed all the functionality inside MyAudioSubsystem (Audio Manager) class, and I used the level blueprint classes to call the music playlists on Beginplay()
Important:
Please consider that this guide does not intend to teach creative sound design. Instead, it exclusively focuses on game audio's implementation and programming aspects.
Download the project here:
IMPORTANT!
〰️
IMPORTANT! 〰️
Prerequisites:
Create an empty C++ third-person project called “MyUEProject.”
Download and integrate the Wwise plugin into your project through the Wwise Launcher.
Activate Enable Auto-Defined SoundBanks in the Wwise Project Settings
For this tutorial to make sense, you must know how to integrate the Wwise plugin into Unreal Engine. Additionally, consider reading and referencing my previous post about UPROPERTY & UFUNCTION and How To Create an Audio Manager in Unreal Engine:
IMPORTANT!
〰️
IMPORTANT! 〰️
Wwise Music Sequence
I imported a simple music sequence with two layers (music tracks) for this system. I positioned the post-exit marker in the correct location.
I created a playlist container and set the music segment to loop “Infinite”.
I added a state group with two states, Layer1 and Layer2, and defined a simple voice volume fade on the Layer2 music track when the Layer1 state is set.
Finally, I created an event pointing to the music playlist. I added a short 0.5-second delay so the music doesn’t start immediately when the world is created.
In Unreal Engine, I used the Wwise Browser to refresh, generate soundbanks, and consolidate assets. A new Wwise Event .uasset shows up in the correct folder path.
C++ Implementation
The first step in any C++ implementation is to include all the necessary dependencies on the build.cs file
These are required to compile the project with the Wwise plugin.
MyUEProject.build.cs
Registering the Music & UI, and Listener Objects:
I created a Game Instance Subsystem as my Audio Manager. Using this method, I am sure these new objects will be registered before any world is instanced and not destroyed between level transitions; its functions can be globally accessible.
Allocate new Ak Game Objects:
To include these field types, I added
#include "AkGameObject.h"
MyAudioSubsystem.h
Register Ak Objects on Initialize():
I called the virtual function Initialize() from MyAudioSubsystem and declared a new function to register these new Ak Game Objects called RegisterAkGameObjects(). Additionally, I set these objects' default channel settings to stereo and disabled spatialization.
MyAudioSubsystem.h
To get the Wwise Sound Engine pointer, add
#include "Wwise/API/WwiseSoundEngineAPI.h"
MyAudioSubsystem.cpp
Play And Stop Music:
I created a function to post a provided Ak Audio Event on the newly registered MUSIC_AND_UI_OBJECT.
MyAudioSubsystem.h
MyAudioSubsystem.cpp
Levels and Blueprints
I created two levels. Each one contains a set of Trigger Box actors to call the new audio functions OnActorBeginOverlap()
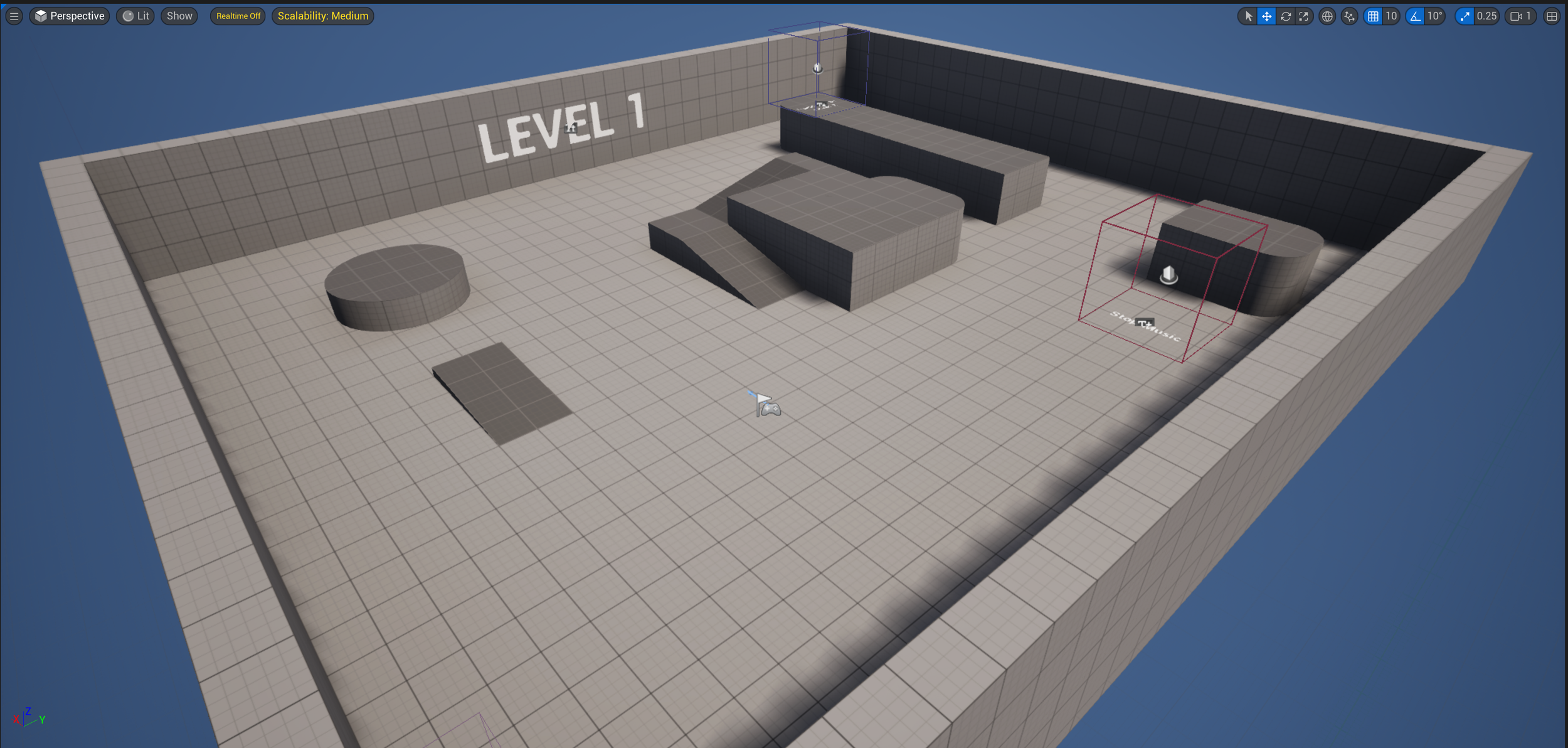

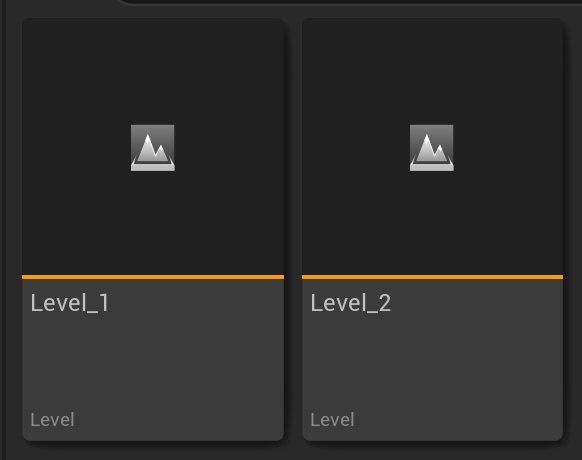
Level Blueprint Implementation:
The level Blueprint has easy access to every actor it contains, so it's an excellent place to prototype this system quickly. To get a reference to the Trigger Boxes, select a box on the viewport, right-click on the level blueprint's event graph, and find all its events on the list.
Transition to Level:
When the player character overlaps with the box, transition to another level
Stop Current Music Event:
When the player character overlaps with the box, call the StopCurrentMusicEvent() on the AudioSubsystem
Play New Music Event:
After the new world (Level) is created, set a new state and play a new music event. PlayNewMusicEvent() is called on the AudioSubsystem and will only post a new event if the provided Event ID differs from the one currently playing.
The Wwise Profiler
Verifying that this system is correctly set up by using the Wwise profiler is easy.
Connect the profiler to the Unreal Editor
Open the Advanced Profiler
The new 2D listener is registered with the Engine and listens to itself and the Music and UI object. The profiler also shows that spatialization is not activated on these objects.
On the Voices Graph tab, it is easy to verify that the music event is posted on the Music and UI object and is being sent to the Listener (Non-World)
What about UI sounds?
UI sounds don’t require as much management as Music events, so posting audio events on the Music and UI object is sufficient.
MyAudioSubsystem.h
MyAudioSubsystem.cpp